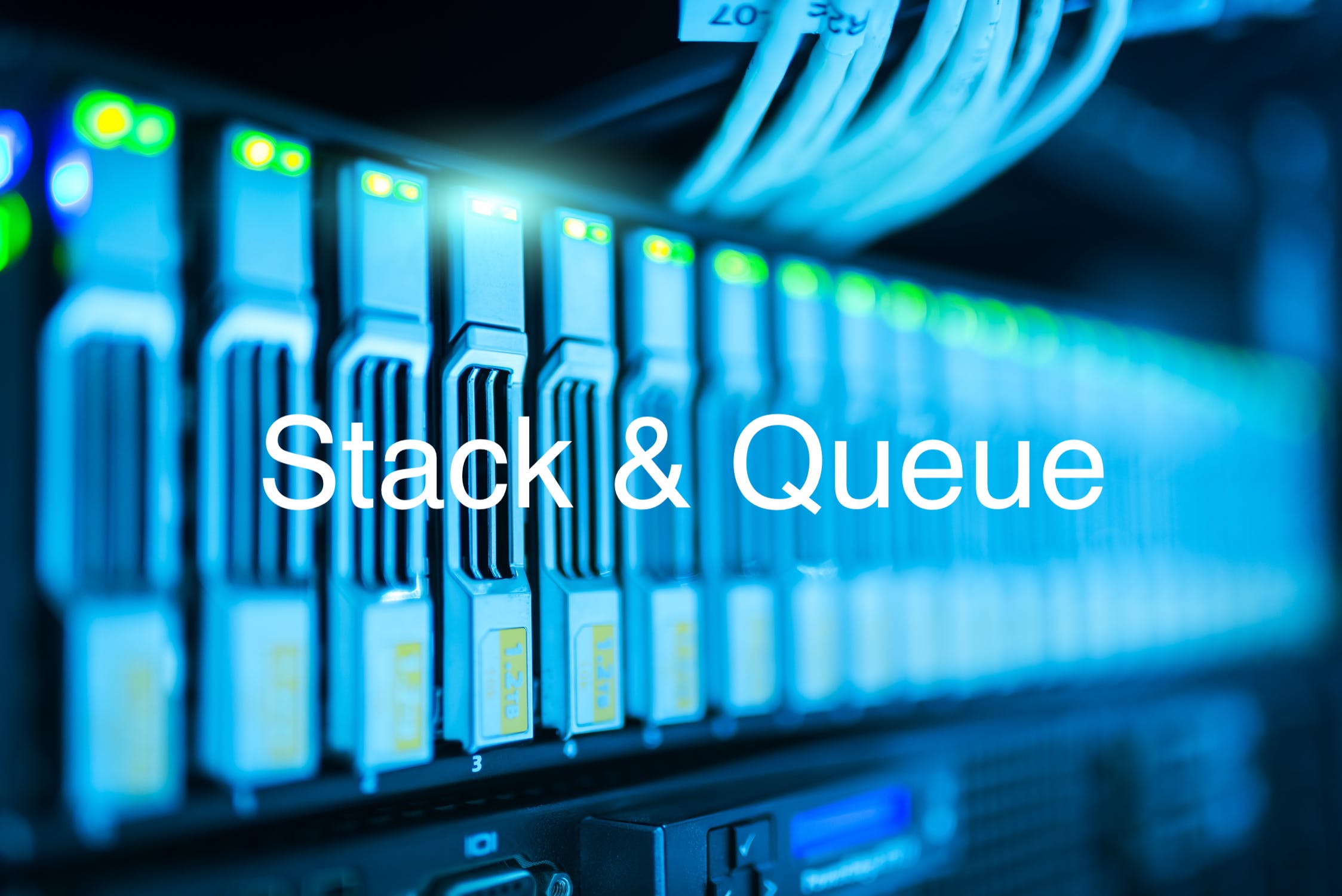
Stack & Queue
當我們碰到大量資料的時候,通常都會用陣列來處理,資料結構中處理陣列有兩種較常見的方式:堆疊(stack)與佇列(queue)。
Stack
Stack 可以想像把盤子疊起來,想要拿出中間的盤子,只能先把最上面的盤子一個個拿走,LIFO
優點:
pop
、push
、peek
: 如果只是對最尾端資料做操作,O(1)
缺點:
lookup
: 要查看資料,要從尾端往前找,O(n)
使用時機 :
Javascript Stack:
Array 實作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Stack { constructor(){ this.array = []; } peek() { return this.array[this.array.length-1]; } push(value){ this.array.push(value); return this; } pop(){ this.array.pop(); return this; } }
|
Linked List 實作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| class Node { constructor(value){ this.value = value; this.next = null; } }
class Stack { constructor(){ this.top = null; this.bottom = null; this.length = 0; } peek() { return this.top; } push(value){ const newNode = new Node(value); if (this.length === 0) { this.top = newNode; this.bottom = newNode; } else { const holdingPointer = this.top; this.top = newNode; this.top.next = holdingPointer; } this.length++; return this; } pop(){ if (!this.top) { return null; } if (this.top === this.bottom) { this.bottom = null; } const holdingPointer = this.top; this.top = this.top.next; this.length--; return this; } }
|
Queue
Queue 可以想像排隊,先來排在最前面的可以先離開,FIFO
優點:
enqueue
、dequeue
、peek
: 如果只是對最前端資料做操作,O(1)
缺點:
lookup
: 要查看某個位置的資料,要從最前端往後找,O(n)
使用時機 :
Javascript queue:
Linked List 實作
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| class Node { constructor(value) { this.value = value; this.next = null; } }
class Queue { constructor(){ this.first = null; this.last = null; this.length = 0; } peek() { return this.first; } enqueue(value){ const newNode = new Node(value); if (this.length === 0) { this.first = newNode; this.last = newNode; } else { this.last.next = newNode; this.last = newNode; } this.length++; return this; } dequeue(){ if (!this.first) { return null; } if (this.first === this.last) { this.last = null; } const holdingPointer = this.first; this.first = this.first.next; this.length--; return this; } }
|
Comments